gazefilter.app is a versatile web application designed for real-time eye-tracking, offering seamless integration capabilities for developers and researchers. This powerful tool can be easily incorporated into various applications, enhancing them with eye-tracking functionality.
GazeFilter application provides web-based eye-tracking with these core features:
- Local Processing: Video stream is processed on-device, ensuring privacy and lowest latency for real-time tracking.
- Resource Efficiency: Utilizes classical machine learning and computer vision algorithms for optimal performance, avoiding heavy deep learning models.
- Web Embeddable: Easily integrate eye-tracking into your applications by running it in a separate browser context.
Gazefilter aims to provide broad compatibility across different browsers and operating systems. The table below illustrates the current compatibility status:
Platform | Chrome | Edge | Firefox | Safari |
Windows | ✅ | ✅ | ✅ | ➖ |
Mac | ✅ | ✅ | ✅ | ✅ |
Linux | ❔ | ❔ | ❔ | ➖ |
Android | ✅ | ✅ | ✅ | ➖ |
iOS | ✅ | ❔ | ✅ | ✅ |
✅ — compatible, 🚧 — under development, ❔ — unknown, ❌ — not compatible, ➖ — browser not available
gazefilter.app can be used as an overlay with gaze visualization in OBS Studio without requiring any plugins or additional software. You just need to do a little setup to get it working seamlessly.
To begin, launch OBS with the additional CLI parameter --enable-media-stream
. This parameter is essential for enabling the Browser Source in OBS to utilize media devices.
-
Windows using PowerShell:
Start-Process "obs64.exe" -ArgumentList "--enable-media-stream" -WorkingDirectory "C:\Program Files\obs-studio\bin\64bit"
-
Windows Command Prompt:
cd /d "C:\Program Files\obs-studio\bin\64bit" && obs64.exe --enable-media-stream
For convinience, you can add this parameter to the “Target” field at the end (after "
) in the shortcut properties on Windows.
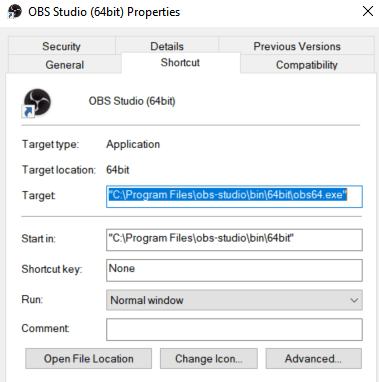
Enabling the --enable-media-stream
parameter allows any Browser Source you add in OBS to access media devices such as your camera and microphone. Ensure that you trust any other site you use as a Browser Source to avoid potential privacy and security risks.
Next, create a new Browser Source.
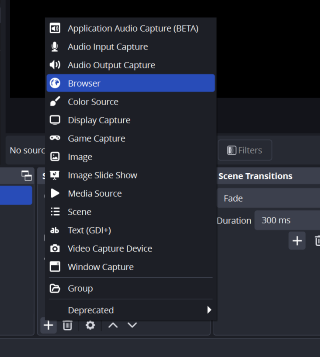
You can name this source as you like, for instance, “Gazefilter Eye Tracker”.
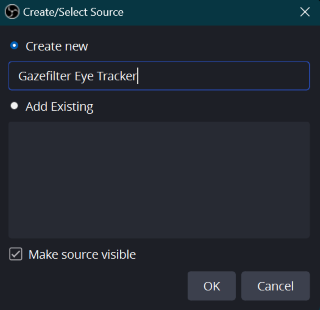
In the properties for the newly created browser source, you’ll need to configure several settings:
-
Set the URL:
https://gazefilter.app?startup=true&calib=full
The startup
parameter ensures that gazefilter attempts to connect to a video device immediately upon loading.
-
Adjust the width and height to match the resolution of the display you’re recording.
For example, if you have two screens with resolutions of 1920x1080 and 2560x1440, and you intend to stream the second one, set the width to 2560 and the height to 1440.
-
Check the Use custom frame rate option and set the FPS to 60.
Even if your camera does not support 60 FPS, it is better to set the custom frame rate to the highest possible value. This setting controls the rendering rate for the web page, and a higher rate can reduce video frame processing drops and make the calibration process smoother.
-
Add the following custom CSS:
body { background-color: transparent; }
Note: This custom CSS makes the Gazefilter appear as a transparent overlay, allowing it to blend with your OBS scene.
-
Optionally, if you prefer Gazefilter to disconnect from a device when source is not visible, check the Shutdown source when not visible option.
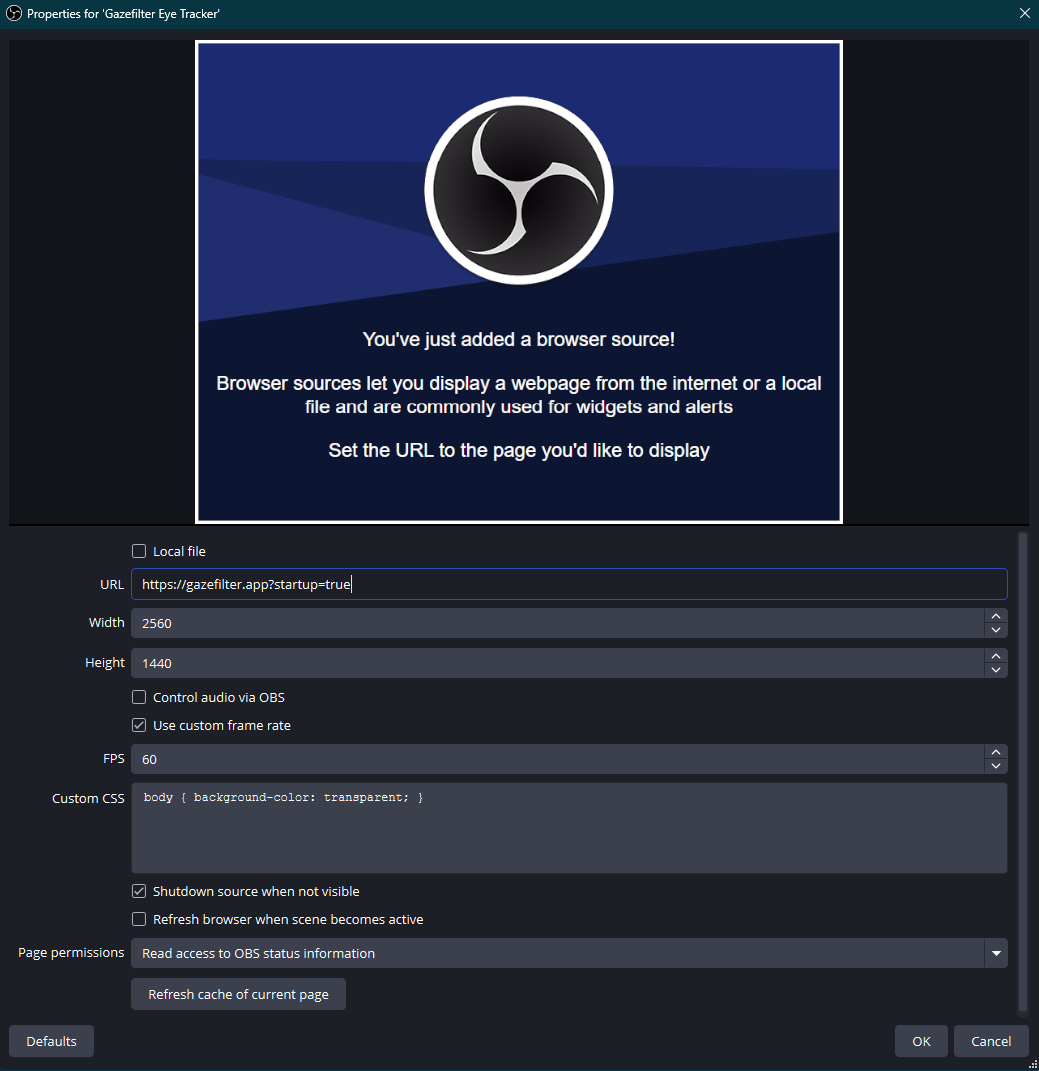
Leave all other parameters at their default settings and click OK.
If your screen resolution does not match the recording output size (for instance, if your screen has a 2K resolution but OBS output is Full HD), you can fit the browser source to the recording output by right-clicking on the browser source, selecting Transform → Fit to Screen, or by selecting the browser source and pressing Ctrl+F
.
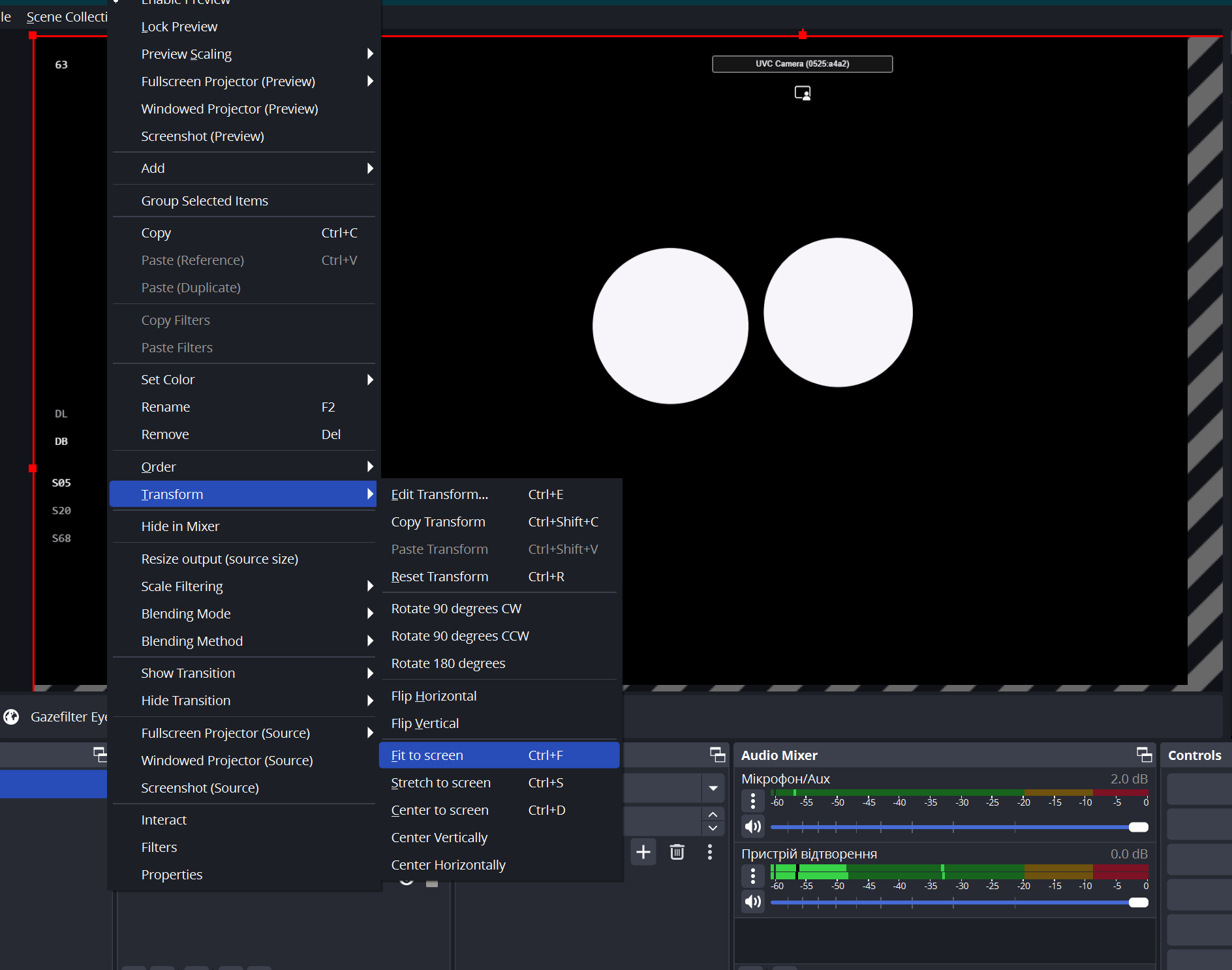
To fine-tune eye settings such as detection models, device selection, and calibration, you’ll need to interact directly with the web page within OBS. Select the browser source with gazefilter and click Interact.
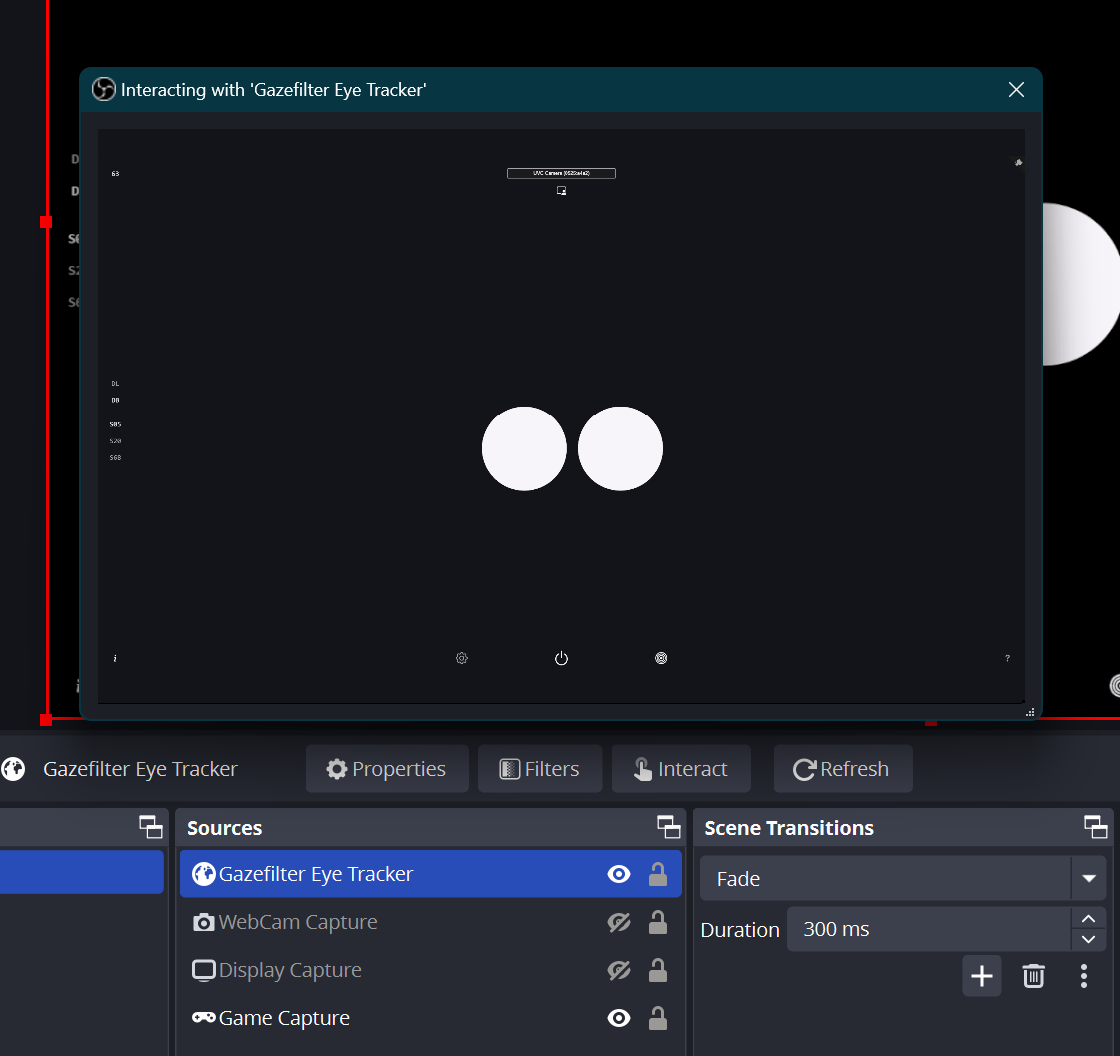
This will open a new window where you can interact with the gazefilter UI.
If multiple video devices are available, you can select the desired device by pressing the digit key from 1
to 0
on your keyboard, where 1
is the first device.
Keep this interaction window open for the subsequent steps.
For optimal eye-tracking performance:
- ensure your camera is positioned at eye level near the screen you want to use for gaze overlay,
- use the application in a well-lit environment, ideally with the light source positioned behind the camera,
- the screen should be within a hand’s reach,
- if you wear glasses, make sure that reflections on the lenses do not obstruct the visibility of your pupils.
You can try the calibration at gazefilter.app in the web browser of your choice to test the gaze calibration. The workflow there will be the same.
Before enabling a gaze overlay, you need to perform gaze calibration. To do this, Gazefilter should be rendered over the screen that OBS is capturing. Right-click on the browser source with gazefilter and select Fullscreen Projector → [display name].
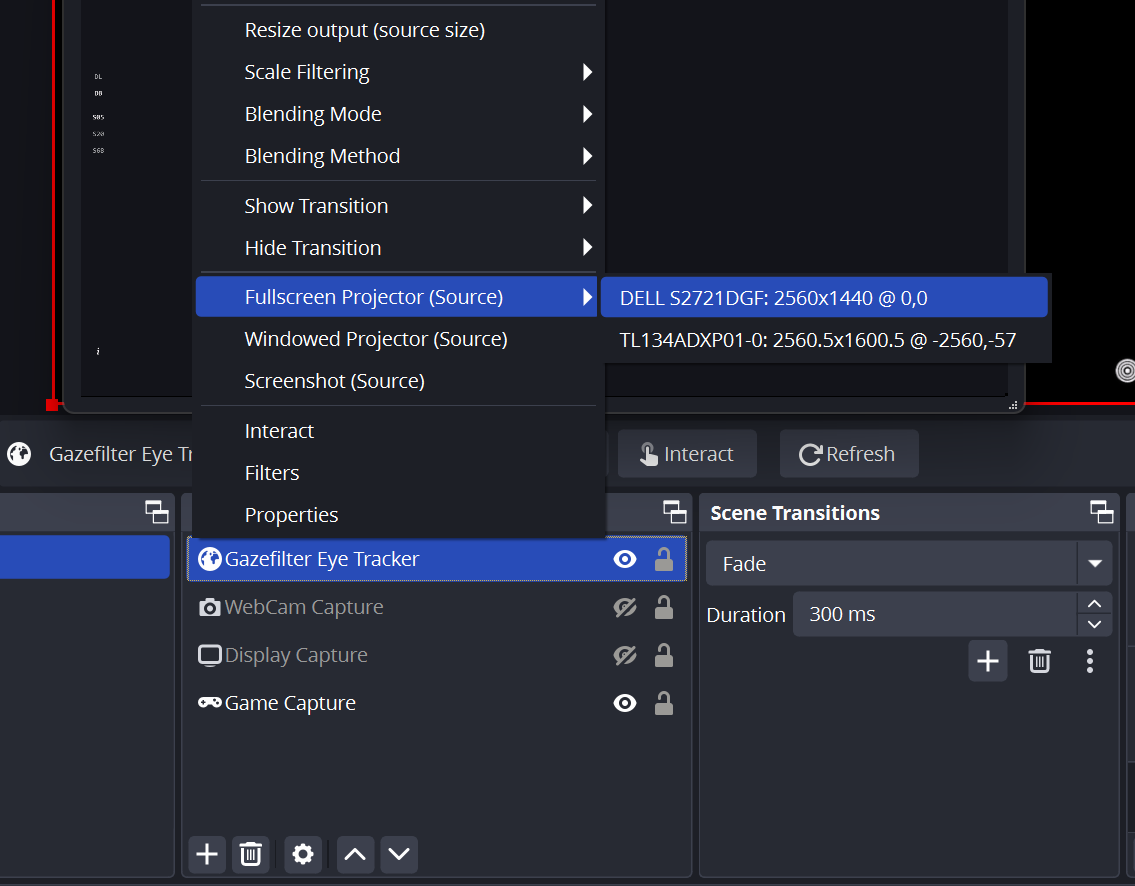
In the interaction window, click the calibration button (located to the right of the power button) and follow the instructions on the projected window. Ensure that the interaction window is in focus for key-based interactions during calibration.
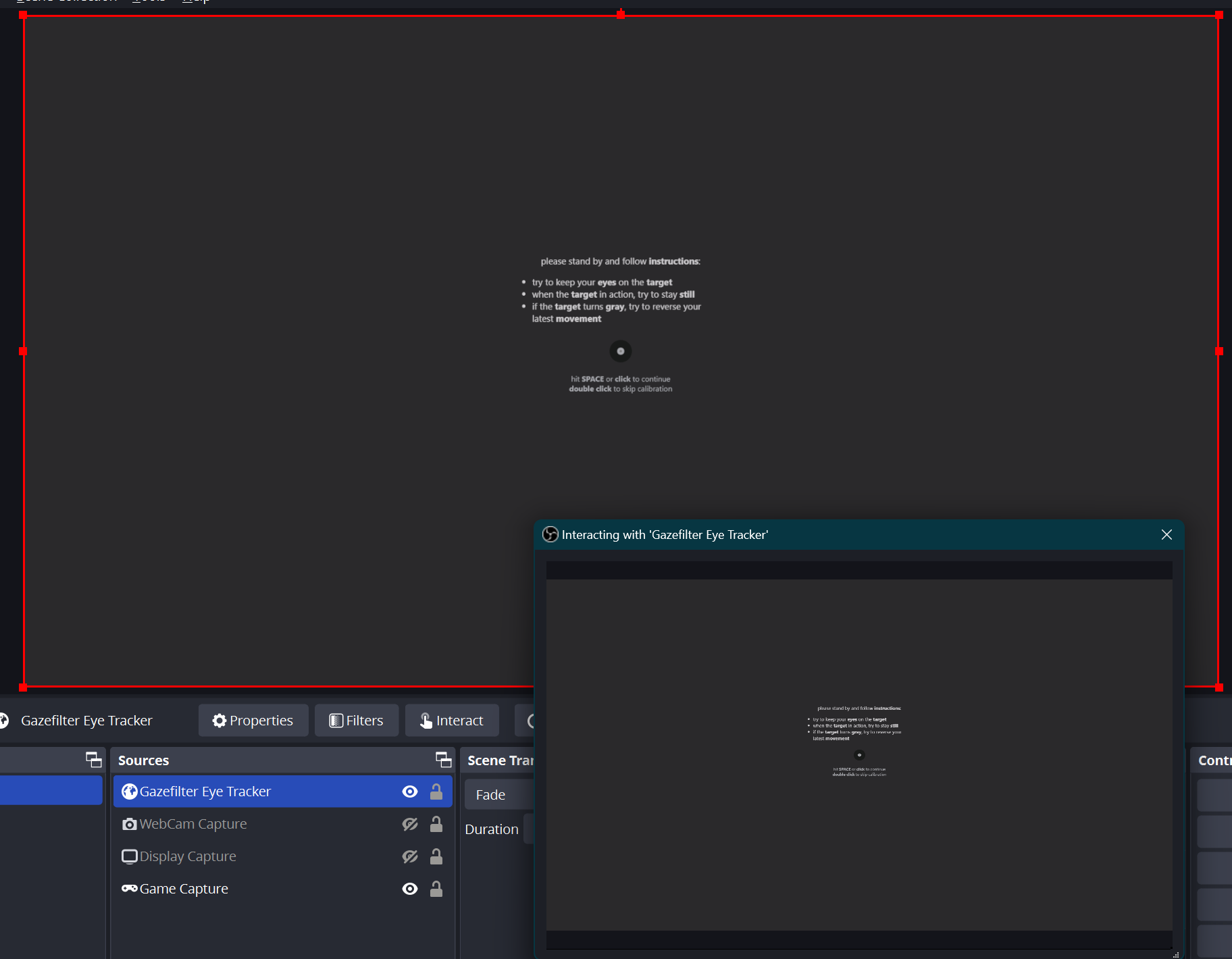
Ensure you look at the screen where the Fullscreen Projector window is displayed, not the OBS or browser source interaction window.
Once calibration is complete, you should see a thin circle moving on the screen, which visualizes your gaze. You can close the projection window.
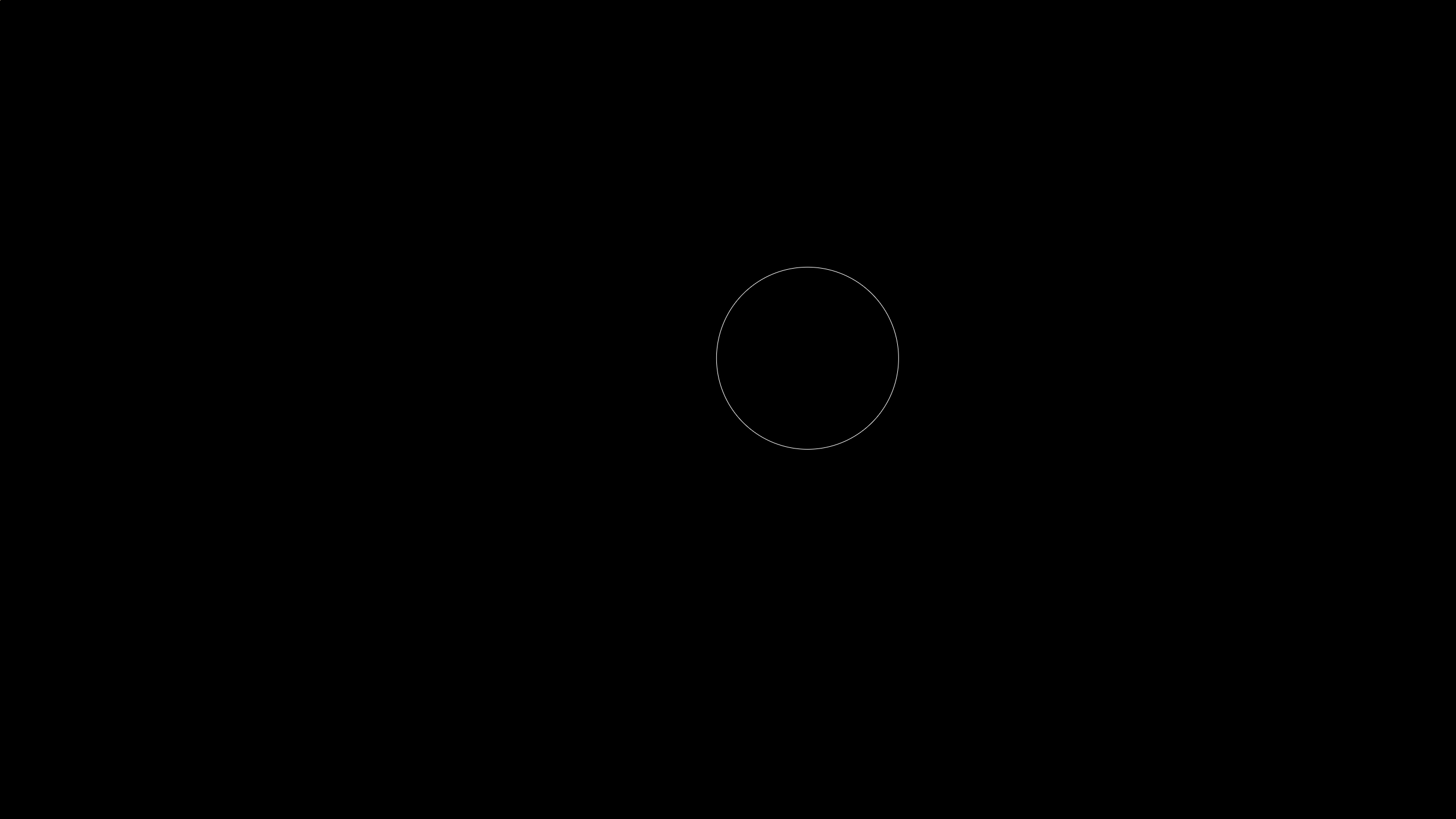
This overlay will now be visible in the OBS recording preview. Make sure that the browser source is positioned above other opaque sources in the source list.
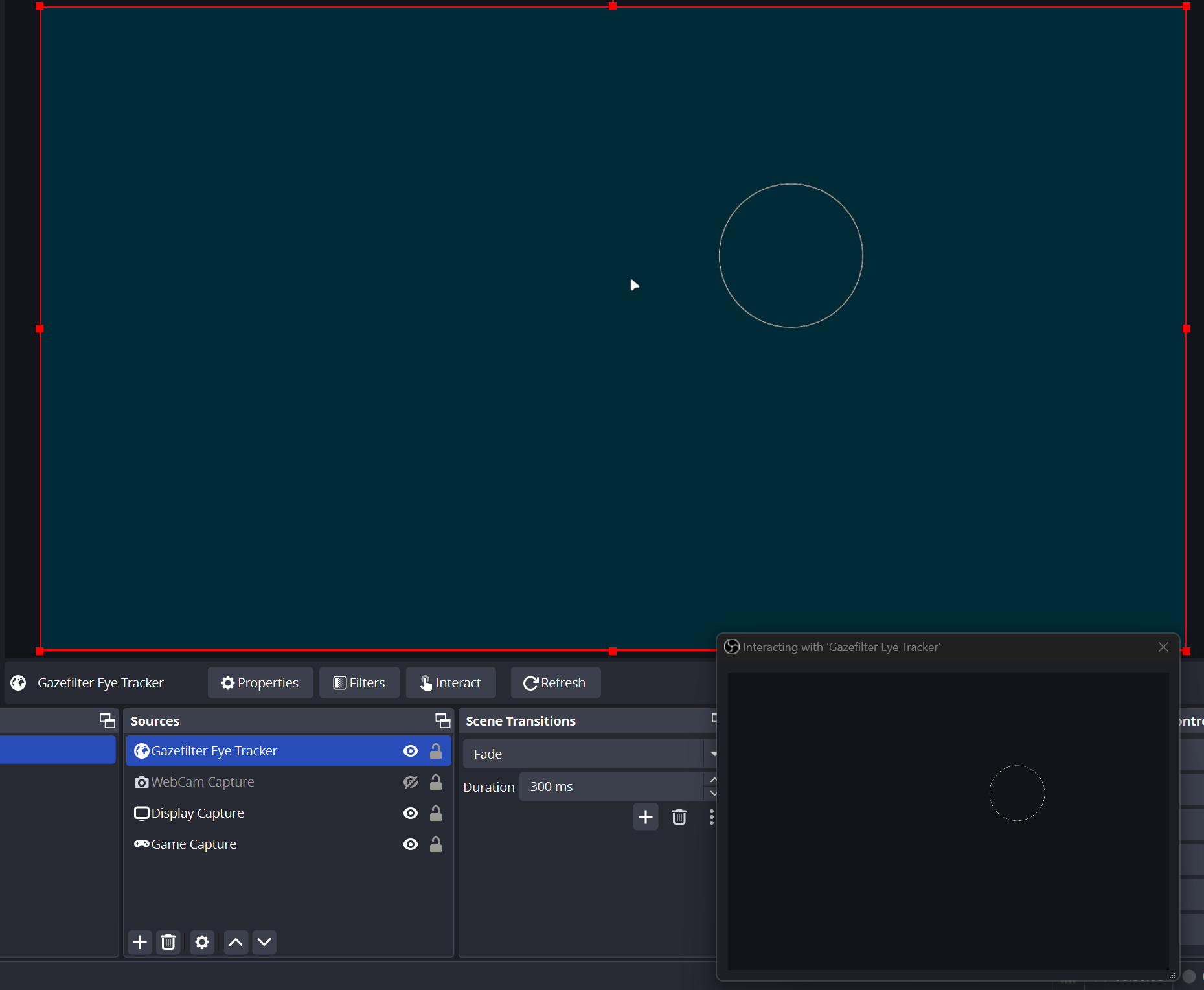
Calibration is saved automatically. To open the gaze overlay without going through the calibration process again, start calibration and then double-click inside the interaction window.
All is set up! You can now stream or record with the active gaze overlay.
To integrate Gazefilter into your web application using plain JavaScript and HTML, you need to:
- Add an
<iframe />
element pointing to gazefilter.app
- Handle the initial message from the iframe containing a
MessagePort
- Add a message handler to the message port for ongoing communication
Let’s go through each step in detail.
Add the following HTML code where you want the gazefilter interface to appear:
<iframe
src="https://gazefilter.app"
width="640"
height="480"
allowfullscreen
allow="camera">
</iframe>
Explanation of the attributes:
-
src
: The URL of the gazefilter application. See available parameters for advanced initialization.
-
allow="camera"
: This mandatory attribute grants the iframe permission to access the user’s camera, which is necessary for the gazefilter’s functionality.
-
(optional) width
and height
: These set the size of the iframe. See interface behaviour for details.
-
(optional) allowfullscreen
: Necessary if gaze calibration need to be started by a user gesture requiring fullscreen mode.
Add an event listener to handle the initial message:
// initialize placeholder variable for message port
let port = null;
window.addEventListener('message', event => {
// check if the current event comes from gazefilter
if (event.origin !== 'https://gazefilter.app') return;
// check channel event which holds a message port
if (event.data.type === 'channel') {
// close previous message port
if (port !== null) port.close();
// set message port
port = event.ports[0];
port.onmessage = ongazefilter; // this function is described in the next step
}
});
Implement the function to handle incoming events from the message port:
function ongazefilter(event) {
const data = event.data;
switch (data.type) {
case 'capture':
console.log('Gazefilter capture:', data);
break;
case 'interface':
console.log('Gazefilter mode:', data.interfaceMode);
break;
case 'connect':
console.log('Gazefilter connected ', data.deviceLabel);
break;
case 'dispose':
console.log('Gazefilter disconnected from camera');
break;
// Add more cases for other message types as needed
}
}
This basic setup allows to receive and handle messages from the eye-tracker. The message handler can be expanded to process other types of messages. See Gazefilter Events for detailed description.
For a full working example following this guide you can check out this CodePen.
GazeFilter’s interface automatically adapts based on viewport dimensions.
The default interface mode shows the full set of controls and video preview. Initialization parameters can be used to override which controls are visible (see initialization parameters).
Tracker Mode is active when neither Status Mode nor Calibration Mode conditions are met.
When the viewport becomes extremely small, the interface switches to Status Mode. This mode shows only minimal status indicators — a single eye icon when tracking and double X icons when not tracking. Video preview and controls are hidden.
Triggers when:
- width ≤ 100px OR height ≤ 50px.
In Calibration Mode, the interface hides most UI elements and shows only the calibration procedure. This mode is designed for precise eye-tracking calibration with minimal distractions.
Triggers when any of these conditions are met:
- fullscreen mode is active on the container element,
- calibration is explicitly requested but fullscreen API fails,
- the viewport size approaches screen resolution.
The viewport size check behaves differently depending on context:
- In embedded contexts (iframes, etc.): Triggers if EITHER width OR height matches screen resolution
- In standalone contexts: Requires a more strict match of dimensions to screen resolution
Calibration can be initiated in several ways:
- Calibration Button: Clicking the calibration button first attempts to use the Fullscreen API.
- Automatic Fallback: If the fullscreen request fails (common in iOS browsers or restricted environments), the application automatically sets an internal override flag to enter calibration mode without requiring fullscreen.
- Size-based Detection: The interface automatically switches to calibration mode when the viewport size approaches the screen resolution according to the embedded/non-embedded rules above.
The calibration mode can be exited by:
- Pressing the Escape key (exits both fullscreen and override modes)
- Using the browser’s built-in fullscreen exit controls (which the application detects)
When loading gazefilter.app, you can use several query parameters to customize the initialization behavior of the application.
The startup
parameter overrides the default behavior for camera connection on application launch.
By default, gazefilter.app does not attempt to connect to a camera automatically when launched for the first time. However, on subsequent launches, if there was a successful camera connection in a previous session, the application will attempt to connect to the most recently used camera.
Values:
true
— attempts to connect to a camera even on first launch,
false
— never attempts to connect to a camera automatically.
The origin
parameter provides an extra layer of security by restricting the Gazefilter communicate only with the specified origin.
The vfp
parameter enables polyfilling for Video Frame API, even if the browser supports it.
Can be useful when the browser’s implementation of the Video Frames API has known bugs or issues.
The controls
parameter customizes which UI control elements are visible.
Values:
none
— hides all controls
full
or all
— shows all controls (default)
- Comma-separated list of controls to show:
devices
— camera device selection
models
— model selection
preview
— preview settings
hints
— toggle for UI hints
power
— power toggle
settings
— settings and calibration
help
— question mark button
The visuals
parameter allows tweaking UI elements visibility.
Values (comma-separated):
nocorner
— hides the GitHub corner link
nofps
— hides the frame rate counter
The config
parameter allows customizing the Gazefilter configuration. It accepts either a JSON string or a preset identifier.
Use predefined configurations with simple preset identifiers. You can specify either a detector or shape model preset alone, or combine them:
- Detector presets (use alone or as prefix):
DB
— basic detector,
DL
— lightweight detector.
- Shape model presets (use alone or as suffix):
S05
— 5-points face shape model,
S20
— 20-points face shape model,
S68
— 68-points face shape model.
When combining detector and shape model presets:
- Face detector (DB/DL) must always come first,
- Use a hyphen (-) as separator,
- Add shape model (S05/S20/S68) second.
Examples:
DL
— Light detector only,
S20
— 20-points shape model only,
DL-S20
— Light detector with 20-points shape model.
For advanced customization, provide a JSON string with configuration overrides:
{
"backend": {
"face_shaper": "models/face-05.shaper.bin",
"face_pinmap": "models/face-05.shaper.json",
"core": {
"pose": {
"keys": [
"nose_bottom",
"left_eye_outer_corner",
"right_eye_outer_corner",
"left_eye_inner_corner",
"right_eye_inner_corner"
]
}
}
}
}
When passing JSON configuration in URL parameters, use a minified string without newlines.
The calib
parameter allows customizing the calibration sequence. It accepts either a preset identifier or a custom sequence definition.
Preset descriptions:
-
quick
— A balanced sequence that requires minimal head movement:
- Initial demo point with introduction
- Classic 9-point calibration on gray background
- Comfort break and closer position calibration
- Rectangle pattern calibration on gray background
- Comfort break and farther position calibration
- Classic 9-point calibration on gray background
- Perimeter pattern on white background
- Rectangle pattern on black background
- Final outro message
-
basic
— Standard sequence with head movement variations:
- Initial demo point with introduction
- Classic 9-point calibration on gray background
- Left-facing triangle pattern calibration
- Comfort break and right-facing triangle pattern calibration
- Comfort break and closer position rectangle pattern
- Comfort break and farther position classic pattern
- Classic 9-point calibration on white background
- Classic 9-point calibration on black background
- Final outro message
-
long
— Extended sequence with dense patterns:
- Initial demo point with introduction
- Dense pattern calibration on gray background
- Left-facing diamond pattern calibration
- Comfort break and right-facing diamond pattern calibration
- Comfort break and closer position perimeter pattern
- Comfort break and farther position classic pattern
- Dense pattern calibration on white background
- Dense pattern calibration on black background
- Final outro message
-
full
— Comprehensive sequence covering all head positions:
- Initial demo point with introduction
- Dense pattern calibration on gray background
- Left-facing diamond pattern calibration
- Right-facing diamond pattern calibration
- Bottom-facing diamond pattern (head tilted forward)
- Top-facing diamond pattern (head tilted backward)
- Elevated position triangle pattern
- Lower position triangle pattern
- Left-tilted triangle pattern at top-left
- Right-tilted triangle pattern at top-right
- Body-left triangle pattern
- Body-right triangle pattern
- Close-range perimeter pattern
- Classic pattern from normal distance
- Dense pattern on white background
- Dense pattern on black background
- Final outro message
For custom sequences, each step is defined using this format:
[background][.calibset][~directive]
Where:
background
: white
, gray
, black
, or interface
(default)
calibset
: empty
(default), demo
, classic
, perimeter
, rectangle
, dense
, diamond
directive
: Instruction like intro
, straight
, comfort
, outro
Important: Point samples are only recorded to the calibration dataset when a non-default background is specified (white
, gray
, or black
). Using the default interface
background or no background will show the points but won’t record samples.
Position values for patterns and single points:
- Cardinal:
top
, right
, bottom
, left
- Diagonal:
right-top
, right-bottom
, left-bottom
, left-top
Available directives:
intro
— initial instructions
straight
— request to face straight ahead
tilt-left
— request to tilt head left
tilt-right
— request to tilt head right
tilt-forward
— request to move chin down
tilt-backward
— request to move chin up
turn-left
— request to turn head left
turn-right
— request to turn head right
elevate
— request to move body up
descent
— request to move body down
move-left
— request to move body left
move-right
— request to move body right
move-closer
— request to move closer to screen
move-farther
— request to move away from screen
comfort
— request to return to comfortable position
outro
— completion message
Some examples of custom sequences:
?calib=demo~intro>white.classic>~outro
?calib=demo~intro>gray.dense>~outro
?calib=demo~intro>gray.rectangle>white.classic>black.diamond>~outro
This creates a sequence with:
- Demo point with intro message
- Classic 9-point calibration on white background
- Final outro message
You can combine multiple parameters using the &
symbol:
https://gazefilter.app?origin=https://example.com&startup=true&controls=devices,models&vpf
This initialization URL will:
- Attempt to connect to a camera on startup
- Restrict communication to
https://example.com
- Show only the device selection and model selection controls
- Enable Video Frame API polyfilling
Title: GazefilterEvent
Description: Events from gazefilter.app.
JSON schema source for the events is available at gazefilter.app/event.schema.json.
1. Property GazefilterChannelEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterChannelEvent |
Description: Handshake event. Sent once the app is ready to communicate.
1.1. [Optional] Property type
Description: Type of the event.
Specific value: "channel"
1.2. [Required] Property timeOrigin
| |
Type | number |
Required | Yes |
Format | double |
Description: Timestamp of the web application initialization.
See performance.timeOrigin.
1.3. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
2. Property GazefilterInterfaceEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterInterfaceEvent |
Description: Interface type change event.
2.1. [Optional] Property type
Description: Type of the event.
Specific value: "interface"
2.2. [Required] Property interfaceMode
| |
Type | enum (of string) |
Required | Yes |
Defined in | #/$defs/InterfaceMode |
Description: Updated interface mode.
Must be one of:
- “status”
- “tracker”
- “calibration”
2.3. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
3. Property GazefilterFocusEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterFocusEvent |
Description: Browser focus event. Sent every time window is focused or blurred.
3.1. [Optional] Property type
Description: Type of the event.
Specific value: "focus"
3.2. [Required] Property hasFocus
Description: Whether the application window has focus.
See document.hasFocus.
3.3. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
4. Property GazefilterVisibilityEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterVisibilityEvent |
Description: Document visibility change event. Sent every time the document is hidden or shown.
4.1. [Optional] Property type
Description: Type of the event.
Specific value: "visibility"
4.2. [Required] Property visibilityState
| |
Type | enum (of string) |
Required | Yes |
Defined in | #/$defs/VisibilityState |
Description: Current visibility state of the document.
See document.visibilityState.
Must be one of:
- “unknown”
- “hidden”
- “visible”
4.3. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
5. Property GazefilterConfigEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterConfigEvent |
Description: Gazefilter configuration update event.
5.1. [Optional] Property type
Description: Type of the event.
Specific value: "config"
5.2. [Required] Property gazefilterConfig
| |
Type | object |
Required | Yes |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterConfig |
Description: Updated gazefilter configuration.
5.2.1. [Optional] Property user
5.2.1.1. Property UserConfig
5.2.1.1.1. [Optional] Property face_model
5.2.1.1.1.1. Property FaceModel
| |
Type | object |
Required | No |
Additional properties | [Should-conform] |
Defined in | #/$defs/FaceModel |
5.2.1.1.1.1.1. Property additionalProperties
| |
Type | array of number |
Required | No |
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.1.1.1.1.1.1. additionalProperties items
| |
Type | number |
Required | No |
Format | float |
5.2.1.1.1.2. Property item 1
5.2.1.1.2. [Optional] Property vision
5.2.1.1.2.1. Property Vision
5.2.1.1.2.1.1. Property item 0
5.2.1.1.2.1.1.1. [Required] Property type
Specific value: "binocular"
5.2.1.1.2.1.2. Property item 1
5.2.1.1.2.1.2.1. [Required] Property type
Specific value: "monocular"
5.2.1.1.2.1.2.2. [Required] Property side
5.2.1.1.2.1.2.2.1. Property item 0
Description: Left side.
Specific value: "left"
5.2.1.1.2.1.2.2.2. Property item 1
Description: Right side.
Specific value: "right"
5.2.1.1.2.2. Property item 1
5.2.1.2. Property item 1
5.2.2. [Optional] Property camera
5.2.2.1. Property CameraConfig
5.2.2.1.1. [Optional] Property intrinsics
5.2.2.1.1.1. Property CameraIntrinsicsConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/CameraIntrinsicsConfig |
5.2.2.1.1.1.1. [Optional] Property focals
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.2.1.1.1.1.1. focals item 0
| |
Type | number |
Required | No |
Format | double |
5.2.2.1.1.1.1.2. focals item 1
| |
Type | number |
Required | No |
Format | double |
5.2.2.1.1.1.2. [Optional] Property principal
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.2.1.1.1.2.1. principal item 0
| |
Type | number |
Required | No |
Format | double |
5.2.2.1.1.1.2.2. principal item 1
| |
Type | number |
Required | No |
Format | double |
5.2.2.1.1.1.3. [Optional] Property skew
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.2.1.1.2. Property item 1
5.2.2.1.2. [Optional] Property specs
5.2.2.1.2.1. Property CameraSpecificationConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/CameraSpecificationConfig |
5.2.2.1.2.1.1. [Optional] Property pixels
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.2.1.2.1.1.1. pixels item 0
| |
Type | integer |
Required | No |
Format | uint |
5.2.2.1.2.1.1.2. pixels item 1
| |
Type | integer |
Required | No |
Format | uint |
5.2.2.1.2.1.2. [Optional] Property dimensions
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.2.1.2.1.2.1. dimensions item 0
| |
Type | number |
Required | No |
Format | double |
5.2.2.1.2.1.2.2. dimensions item 1
| |
Type | number |
Required | No |
Format | double |
5.2.2.1.2.2. Property item 1
5.2.2.1.3. [Optional] Property fps
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.2.1.4. [Optional] Property fov
5.2.2.1.4.1. Property FieldOfView
| |
Type | combining |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/FieldOfView |
Description: Camera Field of View in degrees.
5.2.2.1.4.1.1. Property item 0
5.2.2.1.4.1.1.1. [Required] Property horizontal
| |
Type | number |
Required | Yes |
Format | double |
5.2.2.1.4.1.2. Property item 1
5.2.2.1.4.1.2.1. [Required] Property vertical
| |
Type | number |
Required | Yes |
Format | double |
5.2.2.1.4.1.3. Property item 2
5.2.2.1.4.1.3.1. [Required] Property diagonal
| |
Type | number |
Required | Yes |
Format | double |
5.2.2.1.4.2. Property item 1
5.2.2.2. Property item 1
5.2.3. [Optional] Property screen
5.2.3.1. Property ScreenConfig
5.2.3.1.1. [Optional] Property offset
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.3.1.1.1. offset item 0
| |
Type | integer |
Required | No |
Format | int32 |
5.2.3.1.1.2. offset item 1
| |
Type | integer |
Required | No |
Format | int32 |
5.2.3.1.2. [Optional] Property resolution
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.3.1.2.1. resolution item 0
| |
Type | integer |
Required | No |
Format | uint32 |
5.2.3.1.2.2. resolution item 1
| |
Type | integer |
Required | No |
Format | uint32 |
5.2.3.1.3. [Optional] Property pixel
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.3.1.3.1. pixel item 0
| |
Type | number |
Required | No |
Format | float |
5.2.3.1.3.2. pixel item 1
| |
Type | number |
Required | No |
Format | float |
5.2.3.2. Property item 1
5.2.4. [Optional] Property core
5.2.4.1. Property CoreConfig
5.2.4.1.1. [Optional] Property seed
| |
Type | integer or null |
Required | No |
Format | uint64 |
Default | null |
5.2.4.1.2. [Optional] Property face
5.2.4.1.2.1. Property FaceTrackerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/FaceTrackerConfig |
5.2.4.1.2.1.1. [Optional] Property tolerance
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.2. [Optional] Property threshold
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.3. [Optional] Property detect
5.2.4.1.2.1.3.1. Property DetectConfig
5.2.4.1.2.1.3.1.1. [Optional] Property multiscaler
5.2.4.1.2.1.3.1.1.1. Property MultiscalerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/MultiscalerConfig |
5.2.4.1.2.1.3.1.1.1.1. [Optional] Property min_size
| |
Type | integer or null |
Required | No |
Format | uint32 |
Default | null |
5.2.4.1.2.1.3.1.1.1.2. [Optional] Property max_size
| |
Type | integer or null |
Required | No |
Format | uint32 |
Default | null |
5.2.4.1.2.1.3.1.1.1.3. [Optional] Property shift_factor
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.3.1.1.1.4. [Optional] Property scale_factor
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.3.1.1.2. Property item 1
5.2.4.1.2.1.3.1.2. [Optional] Property padding
5.2.4.1.2.1.3.1.2.1. Property PaddingConfig
5.2.4.1.2.1.3.1.2.1.1. [Optional] Property top
| |
Type | integer or null |
Required | No |
Format | int32 |
Default | null |
5.2.4.1.2.1.3.1.2.1.2. [Optional] Property left
| |
Type | integer or null |
Required | No |
Format | int32 |
Default | null |
5.2.4.1.2.1.3.1.2.1.3. [Optional] Property right
| |
Type | integer or null |
Required | No |
Format | int32 |
Default | null |
5.2.4.1.2.1.3.1.2.1.4. [Optional] Property bottom
| |
Type | integer or null |
Required | No |
Format | int32 |
Default | null |
5.2.4.1.2.1.3.1.2.2. Property item 1
5.2.4.1.2.1.3.1.3. [Optional] Property clusterizer
5.2.4.1.2.1.3.1.3.1. Property ClusterizerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/ClusterizerConfig |
5.2.4.1.2.1.3.1.3.1.1. [Optional] Property intersection_threshold
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.3.1.3.1.2. [Optional] Property score_threshold
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.3.1.3.2. Property item 1
5.2.4.1.2.1.3.2. Property item 1
5.2.4.1.2.1.4. [Optional] Property redetect
5.2.4.1.2.1.4.1. Property RedetectConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/RedetectConfig |
5.2.4.1.2.1.4.1.1. [Optional] Property shift_factor
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.4.1.2. [Optional] Property stride_count
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.2.1.4.2. Property item 1
5.2.4.1.2.1.5. [Optional] Property filter
5.2.4.1.2.1.5.1. Property FilterConfig
5.2.4.1.2.1.5.1.1. [Optional] Property beta
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.5.1.2. [Optional] Property mincutoff
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.5.1.3. [Optional] Property dcutoff
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.2.1.5.2. Property item 1
5.2.4.1.2.2. Property item 1
5.2.4.1.3. [Optional] Property pose
5.2.4.1.3.1. Property PoseTrackerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PoseTrackerConfig |
5.2.4.1.3.1.1. [Optional] Property solver
5.2.4.1.3.1.1.1. Property PoseSolverConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PoseSolverConfig |
5.2.4.1.3.1.1.1.1. [Optional] Property consensus
5.2.4.1.3.1.1.1.1.1. Property PoseConsensusConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PoseConsensusConfig |
5.2.4.1.3.1.1.1.1.1.1. [Optional] Property initialization_hypotheses
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.1.1.2. [Optional] Property initialization_blocks
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.1.1.3. [Optional] Property max_candidate_hypotheses
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.1.1.4. [Optional] Property estimations_per_block
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.1.1.5. [Optional] Property block_size
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.1.1.6. [Optional] Property likelihood_ratio_threshold
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.3.1.1.1.1.1.7. [Optional] Property inlier_threshold
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.4.1.3.1.1.1.1.2. Property item 1
5.2.4.1.3.1.1.1.2. [Optional] Property estimator
5.2.4.1.3.1.1.1.2.1. Property PoseEstimatorConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PoseEstimatorConfig |
5.2.4.1.3.1.1.1.2.1.1. [Optional] Property gauss_newton_iterations
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.2.1.2. [Optional] Property rotation_convergence_iterations
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.2.1.3. [Optional] Property rotation_convergence_epsilon
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.4.1.3.1.1.1.2.2. Property item 1
5.2.4.1.3.1.1.1.3. [Optional] Property optimizer
5.2.4.1.3.1.1.1.3.1. Property PoseOptimizerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PoseOptimizerConfig |
5.2.4.1.3.1.1.1.3.1.1. [Optional] Property ftol
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.4.1.3.1.1.1.3.1.2. [Optional] Property xtol
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.4.1.3.1.1.1.3.1.3. [Optional] Property gtol
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.4.1.3.1.1.1.3.1.4. [Optional] Property stepbound
| |
Type | number or null |
Required | No |
Format | double |
Default | null |
5.2.4.1.3.1.1.1.3.1.5. [Optional] Property patience
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.3.1.1.1.3.1.6. [Optional] Property scale_diag
| |
Type | boolean or null |
Required | No |
Default | null |
5.2.4.1.3.1.1.1.3.2. Property item 1
5.2.4.1.3.1.1.2. Property item 1
5.2.4.1.3.1.2. [Optional] Property filter
5.2.4.1.3.1.2.1. Property PoseFilterConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PoseFilterConfig |
5.2.4.1.3.1.2.1.1. [Optional] Property translation
5.2.4.1.3.1.2.1.1.1. Property KalmanFilter3DConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/KalmanFilter3DConfig |
5.2.4.1.3.1.2.1.1.1.1. [Optional] Property process_error_covariance
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.3.1.2.1.1.1.2. [Optional] Property measurement_error_covariance
| |
Type | number or null |
Required | No |
Format | float |
Default | null |
5.2.4.1.3.1.2.1.1.2. Property item 1
5.2.4.1.3.1.2.1.2. [Optional] Property rotation
5.2.4.1.3.1.2.1.2.1. Property KalmanFilter3DConfig
5.2.4.1.3.1.2.1.2.2. Property item 1
5.2.4.1.3.1.2.2. Property item 1
5.2.4.1.3.1.3. [Optional] Property keys
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | N/A |
Max items | N/A |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
FacePin | - |
5.2.4.1.3.1.3.1. FacePin
| |
Type | enum (of string) |
Required | No |
Defined in | #/$defs/FacePin |
Must be one of:
- “nose_bottom”
- “nose_top”
- “nose_tip”
- “left_eye_inner_corner”
- “left_eye_outer_corner”
- “right_eye_inner_corner”
- “right_eye_outer_corner”
- “left_eye_lower_lid”
- “left_eye_upper_lid”
- “right_eye_lower_lid”
- “right_eye_upper_lid”
- “left_mouth_corner”
- “right_mouth_corner”
- “chin_tip”
5.2.4.1.3.2. Property item 1
5.2.4.1.4. [Optional] Property eye
5.2.4.1.4.1. Property EyeTrackerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/EyeTrackerConfig |
5.2.4.1.4.1.1. [Optional] Property scale
| |
Type | number or null |
Required | No |
Format | float |
5.2.4.1.4.2. Property item 1
5.2.4.1.5. [Optional] Property pupil
5.2.4.1.5.1. Property PupilTrackerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PupilTrackerConfig |
5.2.4.1.5.1.1. [Optional] Property localize
5.2.4.1.5.1.1.1. Property LocalizeConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/LocalizeConfig |
5.2.4.1.5.1.1.1.1. [Optional] Property perturbator
5.2.4.1.5.1.1.1.1.1. Property PerturbatorConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/PerturbatorConfig |
5.2.4.1.5.1.1.1.1.1.1. [Optional] Property translate
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.4.1.5.1.1.1.1.1.1.1. translate item 0
| |
Type | number |
Required | No |
Format | float |
5.2.4.1.5.1.1.1.1.1.1.2. translate item 1
| |
Type | number |
Required | No |
Format | float |
5.2.4.1.5.1.1.1.1.1.2. [Optional] Property scale
| |
Type | array or null |
Required | No |
Default | null |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
5.2.4.1.5.1.1.1.1.1.2.1. scale item 0
| |
Type | number |
Required | No |
Format | float |
5.2.4.1.5.1.1.1.1.1.2.2. scale item 1
| |
Type | number |
Required | No |
Format | float |
5.2.4.1.5.1.1.1.1.2. Property item 1
5.2.4.1.5.1.1.1.2. [Optional] Property perturbations
| |
Type | integer or null |
Required | No |
Format | uint |
Default | null |
5.2.4.1.5.1.1.2. Property item 1
5.2.4.1.5.1.2. [Optional] Property filter
5.2.4.1.5.1.2.1. Property FilterConfig
5.2.4.1.5.1.2.2. Property item 1
5.2.4.1.5.2. Property item 1
5.2.4.1.6. [Optional] Property gaze
5.2.4.1.6.1. Property GazeTrackerConfig
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazeTrackerConfig |
5.2.4.1.6.1.1. [Optional] Property threshold
| |
Type | number or null |
Required | No |
Format | float |
5.2.4.1.6.1.2. [Optional] Property tolerance
| |
Type | number or null |
Required | No |
Format | float |
5.2.4.1.6.1.3. [Optional] Property filter
5.2.4.1.6.1.3.1. Property FilterConfig
5.2.4.1.6.1.3.2. Property item 1
5.2.4.1.6.2. Property item 1
5.2.4.2. Property item 1
5.2.5. [Optional] Property backend
5.2.5.1. Property BackendConfig
5.2.5.1.1. [Optional] Property face_detector
| |
Type | string or null |
Required | No |
Default | null |
5.2.5.1.2. [Optional] Property face_shaper
| |
Type | string or null |
Required | No |
Default | null |
5.2.5.1.3. [Optional] Property face_pinmap
| |
Type | string or null |
Required | No |
Default | null |
5.2.5.1.4. [Optional] Property pupil_localizer
| |
Type | string or null |
Required | No |
Default | null |
5.2.5.1.5. [Optional] Property gaze_calibration
| |
Type | string or null |
Required | No |
Default | null |
5.2.5.2. Property item 1
5.3. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
6. Property GazefilterConnectEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterConnectEvent |
Description: Device connect event.
6.1. [Optional] Property type
Description: Type of the event.
Specific value: "connect"
6.2. [Required] Property frameRate
| |
Type | number |
Required | Yes |
Format | double |
Description: Frames per second of the connected device.
See MediaTrackSettings.frameRate.
6.3. [Required] Property frameSize
Description: Frame size in pixels of the connected device as width and height.
See MediaTrackSettings.width
and MediaTrackSettings.height.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
6.3.1. frameSize item 0
| |
Type | integer |
Required | No |
Format | uint32 |
6.3.2. frameSize item 1
| |
Type | integer |
Required | No |
Format | uint32 |
6.4. [Required] Property deviceLabel
Description: Label of the connected device.
See MediaDeviceInfo.label.
6.5. [Optional] Property facingMode
Description: Facing mode of the connected device.
See MediaTrackSettings.facingMode.
6.5.1. Property FacingMode
| |
Type | enum (of string) |
Required | No |
Defined in | #/$defs/FacingMode |
Must be one of:
- “user”
- “environment”
- “left”
- “right”
6.5.2. Property item 1
6.6. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
7. Property GazefilterCaptureEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterCaptureEvent |
Description: Tracking event. Sent every time the new frame is processed.
7.1. [Optional] Property type
Description: Type of the event.
Specific value: "capture"
7.2. [Required] Property presentedFrames
| |
Type | integer |
Required | Yes |
Format | uint32 |
Description: See presentedFrames
in
HTMLVideoElement.requestVideoFrameCallback#metadata
7.3. [Required] Property frameSize
Description: See width
and height
in
HTMLVideoElement.requestVideoFrameCallback#metadata
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.3.1. frameSize item 0
| |
Type | integer |
Required | No |
Format | uint32 |
7.3.2. frameSize item 1
| |
Type | integer |
Required | No |
Format | uint32 |
7.4. [Required] Property presentationTime
| |
Type | number |
Required | Yes |
Format | double |
Description: See presentationTime
in
HTMLVideoElement.requestVideoFrameCallback#metadata
7.5. [Required] Property expectedDisplayTime
| |
Type | number |
Required | Yes |
Format | double |
Description: See expectedDisplayTime
in
HTMLVideoElement.requestVideoFrameCallback#metadata
7.6. [Required] Property mediaTime
| |
Type | number |
Required | Yes |
Format | double |
Description: See mediaTime
in
HTMLVideoElement.requestVideoFrameCallback#metadata
7.7. [Optional] Property processingDuration
| |
Type | number |
Required | No |
Format | double |
Default | null |
Description: See processingDuration
in
HTMLVideoElement.requestVideoFrameCallback#metadata
7.8. [Optional] Property captureTime
| |
Type | number |
Required | No |
Format | double |
Default | null |
Description: See captureTime
in
HTMLVideoElement.requestVideoFrameCallback#metadata
7.9. [Optional] Property trackTime
| |
Type | number |
Required | No |
Format | double |
Default | 0.0 |
Description: Monotonic time in milliseconds since the tracking initialization.
7.10. [Optional] Property frameOffset
| |
Type | array of integer |
Required | No |
Default | [0, 0] |
Description: Difference between camera model frame and processed image in pixels.
Positive values means camera frame is bigger than image,
and negative values means camera frame is smaller than image.
Default values are [0, 0]
and means that camera frame is same as image.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.10.1. frameOffset items
| |
Type | integer |
Required | No |
Format | int32 |
7.11. [Optional] Property frameOrigin
| |
Type | array of integer |
Required | No |
Default | [0, 0] |
Description: Camera model frame center coordinates in pixels.
Denormalize data point to frame coordinates:
(data_point + 1) * frame_origin
.
To convert to image coordinates:
(data_point + 1) * frame_origin + frame_offset
.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.11.1. frameOrigin items
| |
Type | integer |
Required | No |
Format | uint32 |
7.12. [Optional] Property cameraFocal
| |
Type | array of number |
Required | No |
Default | [1.0, 1.0] |
Description: Pinhole camera model focal values in sensor pixel size.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.12.1. cameraFocal items
| |
Type | number |
Required | No |
Format | float |
7.13. [Optional] Property screenOffset
| |
Type | array of integer |
Required | No |
Default | [0, 0] |
Description: Current screen position on virtual screen in pixels.
Default values are [0, 0] and means that current screen is primary.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.13.1. screenOffset items
| |
Type | integer |
Required | No |
Format | int32 |
7.14. [Optional] Property screenOrigin
| |
Type | array of integer |
Required | No |
Default | [0, 0] |
Description: Screen center coordinates in pixels.
Denormalize gaze point to screen coordinates:
- current:
(gaze_point + 1) * screen_origin
,
- virtual:
(gaze_point + 1) * screen_origin + screen_offset
.
Default values are zero and means that screen is not set by the runtime environment.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.14.1. screenOrigin items
| |
Type | integer |
Required | No |
Format | uint32 |
7.15. [Optional] Property screenPixel
| |
Type | array of number |
Required | No |
Default | [1.0, 1.0] |
Description: Screen pixel size.
Default values are [1.0, 1.0].
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.15.1. screenPixel items
| |
Type | number |
Required | No |
Format | float |
7.16. [Optional] Property calibConfidence
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Default | {"left": -1.0, "right": -1.0} |
Defined in | #/$defs/Pair_for_float |
Description: Gaze calibration confidence.
Values are in range 0..1
. In case gaze is not calibrated
values are negative (-1).
7.16.1. [Required] Property left
| |
Type | number |
Required | Yes |
Format | float |
7.16.2. [Required] Property right
| |
Type | number |
Required | Yes |
Format | float |
7.17. [Optional] Property calibOrigin
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0, -1.0] |
Description: Calibration bounding box center in camera space.
If no calibration is performed X and Y values are zero and Z is negative.
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.17.1. calibOrigin items
| |
Type | number |
Required | No |
Format | float |
7.18. [Optional] Property calibSpace
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0, 0.0] |
Description: Half-size of the calibration bounding box in each direction.
If no calibration is performed, values are zero. Use calib_confidence
field for validation.
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.18.1. calibSpace items
| |
Type | number |
Required | No |
Format | float |
7.19. [Optional] Property calibTarget
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0, 0.0] |
Description: Calibration target
Values are [X, Y, W], where X and Y normalized values on screen axes
and W is the weight of the calibration target. Data is not valid if
W is negative.
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.19.1. calibTarget items
| |
Type | number |
Required | No |
Format | float |
7.20. [Optional] Property trackEvent
| |
Type | enum (of integer) |
Required | No |
Default | 0 |
Defined in | #/$defs/TrackEventType |
Description: Track event enumeration.
Possible values are:
-1
- lost,
0
- unknown (default),
1
- found,
2
- tracking.
Properties:
- if greater than 0, the face is detected and tracked,
- if less equal than 0, no face detected or it was lost.
Must be one of:
7.21. [Optional] Property faceConfidence
| |
Type | number |
Required | No |
Format | float |
Default | 0.0 |
Description: Face confidence value.
Negative values means face is not detected.
7.22. [Optional] Property gazeEvent
| |
Type | enum (of integer) |
Required | No |
Default | 0 |
Defined in | #/$defs/GazeEventType |
Description: Gaze event.
Possible values are:
-1
- blink,
0
- unknown (default),
1
- saccade,
2
- fixation,
3
- pursuit.
Must be one of:
7.23. [Optional] Property gazeDuration
| |
Type | number |
Required | No |
Format | double |
Default | 0.0 |
Description: Current gaze event duration on the screen.
Values are in milliseconds and default is zero.
7.24. [Optional] Property gazePoint
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Default | {"left": [0.0, 0.0], "right": [0.0, 0.0]} |
Defined in | #/$defs/Pair_for_Array_size_2_of_float |
Description: Gaze point on the screen.
For denormalization use screen_origin
field values.
Default values are zero. Use gaze_confidence
field for validation.
7.24.1. [Required] Property left
| |
Type | array of number |
Required | Yes |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
left items | - |
7.24.1.1. left items
| |
Type | number |
Required | No |
Format | float |
7.24.2. [Required] Property right
| |
Type | array of number |
Required | Yes |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
right items | - |
7.24.2.1. right items
| |
Type | number |
Required | No |
Format | float |
7.25. [Optional] Property gazeBestPoint
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0] |
Description: Best gaze point on the screen.
Default values are zero. Use gaze_confidence
field for validation.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.25.1. gazeBestPoint items
| |
Type | number |
Required | No |
Format | float |
7.26. [Optional] Property fixationPoint
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0] |
Description: Gaze fixation point on the screen.
Default values are zero. Valid if gaze_event
is fixation.
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.26.1. fixationPoint items
| |
Type | number |
Required | No |
Format | float |
7.27. [Optional] Property facePosition
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0, -1.0] |
Description: Estimated face position in camera 3D space.
Values are in centimeters and coordinate system is right-handed Y-axis down
(X - Right, Y - Down, Z - Forward) from camera looking point.
In case of face not detected or position cannot be estimated, Z value is negative.
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.27.1. facePosition items
| |
Type | number |
Required | No |
Format | float |
7.28. [Optional] Property faceRotation
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0, 0.0] |
Description: Estimated face rotation as Euler angles.
Values are as follows [yaw, pitch, roll]
in radians and coordinate system is
right-handed Y-axis up (X - Right, Y - Up, Z - Back) from users point of view.
Examples:
- zero angles - face is looking towards the camera sensor plane,
- chin goes up - pitch is positive, down - pitch is negative,
- head turns right - yaw is positive, left - yaw is negative,
- head tilts right - roll is positive, left - roll is negative.
In case of face not detected or position cannot be estimated,
values are [0, 0, 0]
. These values are valid only if face_position.z
is positive.
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.28.1. faceRotation items
| |
Type | number |
Required | No |
Format | float |
7.29. [Optional] Property pupilConfidence
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Default | {"left": -1.0, "right": -1.0} |
Defined in | #/$defs/Pair_for_float |
Description: Pupil detection confidence for each eye.
Values are in range 0..1
, and in case of face not detected
or pupil cannot be estimated, values are negative (-1).
7.29.1. [Required] Property left
| |
Type | number |
Required | Yes |
Format | float |
7.29.2. [Required] Property right
| |
Type | number |
Required | Yes |
Format | float |
7.30. [Optional] Property eyeAspectRatio
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Default | {"left": -1.0, "right": -1.0} |
Defined in | #/$defs/Pair_for_float |
Description: Aspect ratio value for each eye.
In case of face not detected or ratio cannot be estimated,
values are negative (-1).
7.30.1. [Required] Property left
| |
Type | number |
Required | Yes |
Format | float |
7.30.2. [Required] Property right
| |
Type | number |
Required | Yes |
Format | float |
7.31. [Optional] Property pupilCenter
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Default | {"left": [0.0, 0.0], "right": [0.0, 0.0]} |
Defined in | #/$defs/Pair_for_Array_size_2_of_float |
Description: Estimated pupil center position on the frame for each eye.
If face is not detected or pupil point cannot be estimated values are [-1, -1]
.
7.31.1. [Required] Property left
| |
Type | array of number |
Required | Yes |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
left items | - |
7.31.1.1. left items
| |
Type | number |
Required | No |
Format | float |
7.31.2. [Required] Property right
| |
Type | array of number |
Required | Yes |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
right items | - |
7.31.2.1. right items
| |
Type | number |
Required | No |
Format | float |
7.32. [Optional] Property eyeTarget
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Default | {"left": [0.0, 0.0, -1.0], "right": [0.0, 0.0, -1.0]} |
Defined in | #/$defs/Pair_for_Array_size_3_of_float |
Description: Tracking regions of interest for each eye.
Describes region of interest for the tracking eyes on the frame as [x, y, size]
.
Negative size
means face is not detected or ROI cannot be estimated.
7.32.1. [Required] Property left
| |
Type | array of number |
Required | Yes |
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
left items | - |
7.32.1.1. left items
| |
Type | number |
Required | No |
Format | float |
7.32.2. [Required] Property right
| |
Type | array of number |
Required | Yes |
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
Each item of this array must be | Description |
right items | - |
7.32.2.1. right items
| |
Type | number |
Required | No |
Format | float |
7.33. [Optional] Property faceTarget
| |
Type | array of number |
Required | No |
Default | [0.0, 0.0, -1.0] |
Description: Tracking face ROI.
Describes region of interest for the tracking face on the frame as [x, y, size]
.
Negative size
means face is not detected.
| Array restrictions |
Min items | 3 |
Max items | 3 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.33.1. faceTarget items
| |
Type | number |
Required | No |
Format | float |
7.34. [Optional] Property faceShape
| |
Type | array of array |
Required | No |
Default | [] |
Description: Face shape points (landmarks) on the frame.
If face is not detected then array is empty.
| Array restrictions |
Min items | N/A |
Max items | N/A |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.34.1. faceShape items
| |
Type | array of number |
Required | No |
| Array restrictions |
Min items | 2 |
Max items | 2 |
Items unicity | False |
Additional items | False |
Tuple validation | See below |
7.34.1.1. faceShape items items
| |
Type | number |
Required | No |
Format | float |
7.35. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
8. Property GazefilterDisposeEvent
| |
Type | object |
Required | No |
Additional properties | [Any type: allowed] |
Defined in | #/$defs/GazefilterDisposeEvent |
Description: Device disconnect event.
8.1. [Optional] Property type
Description: Type of the event.
Specific value: "dispose"
8.2. [Required] Property disposeReason
| |
Type | enum (of string) |
Required | Yes |
Defined in | #/$defs/DisposeReason |
Description: Reason for the device disconnect.
Must be one of:
8.3. [Optional] Property timeStamp
| |
Type | number |
Required | No |
Defined in | #/$defs/EventTimestamp |
Description: Timestamp of the event initialization.
Generated using json-schema-for-humans on 2025-03-03 at 17:15:17 +0000